Analysing Experimental Data in Table Form with Pandas#
There are (as usually the case with python) many different ways and packages to do data analysis and visualisation in python. The package we will look at in this notebook is called Pandas and is probably the most widely used python package for data analysis if the data is in table form. There are many ressources online for free that we encourage you to check out. This notebook will really only scratch the surface of what you can do with pandas.
# Import pandas as pd
import pandas as pd
Loading data file#
The file “data.csv” contains results from a participant who participated in the gaze cue experiment you programmed today!
using .read_csv
we can read in our data file and store it under df
.
url='https://drive.google.com/file/d/1J9mUX7R0mmuQW3-Hwg3W_QNrjbQdzFv7/view?usp=sharing'
url='https://drive.google.com/uc?id=' + url.split('/')[-2]
df = pd.read_csv(url)
df_tutors = pd.DataFrame({
"Name": ["Aylin","Yuri"],
"Age": [27,27]
})
df_tutors
Name | Age | |
---|---|---|
0 | Aylin | 27 |
1 | Yuri | 27 |
type(df)
pandas.core.frame.DataFrame
#Let's look at what df looks like!
df
subject_id | gaze | target | position | congruency | response | rt | correct_response | correct | |
---|---|---|---|---|---|---|---|---|---|
0 | example | left | H | left | congruent | m | 0.471526 | m | 1 |
1 | example | left | F | right | incongruent | y | 0.385041 | y | 1 |
2 | example | right | F | right | congruent | m | 0.315384 | y | 0 |
3 | example | right | H | left | incongruent | y | 0.327810 | m | 0 |
4 | example | right | H | right | congruent | m | 0.319960 | m | 1 |
5 | example | left | F | left | congruent | m | 0.359625 | y | 0 |
6 | example | left | F | left | congruent | y | 0.396527 | y | 1 |
7 | example | left | F | right | incongruent | m | 0.341541 | y | 0 |
8 | example | right | F | right | congruent | y | 0.423950 | y | 1 |
9 | example | left | H | left | congruent | m | 0.325600 | m | 1 |
10 | example | left | H | right | incongruent | y | 0.326414 | m | 0 |
11 | example | right | H | left | incongruent | m | 0.314234 | m | 1 |
12 | example | right | H | right | congruent | y | 0.430067 | m | 0 |
13 | example | right | F | left | incongruent | m | 0.310507 | y | 0 |
14 | example | left | H | right | incongruent | y | 0.394205 | m | 0 |
15 | example | right | F | left | incongruent | m | 0.335534 | y | 0 |
As you can see, we have 9 columns with 15 rows, each row representing a trial. Congruency
refers to whether gaze cue and target position matched in a trial (if yes = congruent, if no = incongruent), the other variables here are pretty self explanatory.
Data frame slicing#
If you want to look at a specific column you can use square brackets and the column name to slice the data frame.
df["response"]
0 m
1 y
2 m
3 y
4 m
5 m
6 y
7 m
8 y
9 m
10 y
11 m
12 y
13 m
14 y
15 m
Name: response, dtype: object
For more information on indexing in pandas: https://pandas.pydata.org/pandas-docs/stable/user_guide/indexing.html
Data frame methods, helper functions#
Pandas has a lot of data frame methods that allow us to do basic analyses.
# run this cell and see what it does
df.describe()
rt | correct | |
---|---|---|
count | 16.000000 | 16.000000 |
mean | 0.361120 | 0.437500 |
std | 0.049647 | 0.512348 |
min | 0.310507 | 0.000000 |
25% | 0.324190 | 0.000000 |
50% | 0.338538 | 0.000000 |
75% | 0.394785 | 1.000000 |
max | 0.471526 | 1.000000 |
Test out different methods below:
# shows the first 3 rows - especially useful with large data frames
df.head(5)
subject_id | gaze | target | position | congruency | response | rt | correct_response | correct | |
---|---|---|---|---|---|---|---|---|---|
0 | example | left | H | left | congruent | m | 0.471526 | m | 1 |
1 | example | left | F | right | incongruent | y | 0.385041 | y | 1 |
2 | example | right | F | right | congruent | m | 0.315384 | y | 0 |
3 | example | right | H | left | incongruent | y | 0.327810 | m | 0 |
4 | example | right | H | right | congruent | m | 0.319960 | m | 1 |
# shows last few rows
df.tail(3)
subject_id | gaze | target | position | congruency | response | rt | correct_response | correct | |
---|---|---|---|---|---|---|---|---|---|
13 | example | right | F | left | incongruent | m | 0.310507 | y | 0 |
14 | example | left | H | right | incongruent | y | 0.394205 | m | 0 |
15 | example | right | F | left | incongruent | m | 0.335534 | y | 0 |
# gives you mean for selected column accuracy
df["correct"].mean()
0.4375
# gives unique entries for column gaze
df["gaze"].unique()
array(['left', 'right'], dtype=object)
# counts number of entries per condition for column gaze
df["gaze"].value_counts()
gaze
left 8
right 8
Name: count, dtype: int64
These helper functions even include some basic plots!
# histogram of accuracy
df["correct"].hist()
<Axes: >
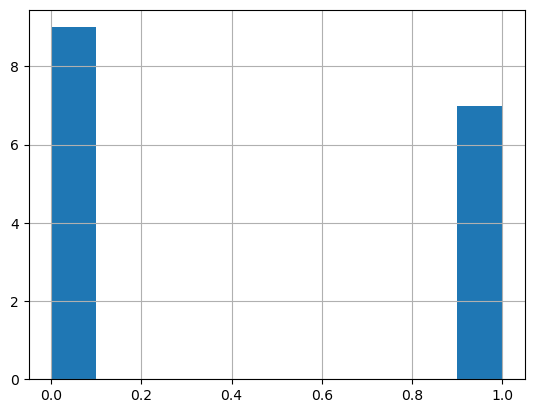
df["rt"].hist()
<Axes: >
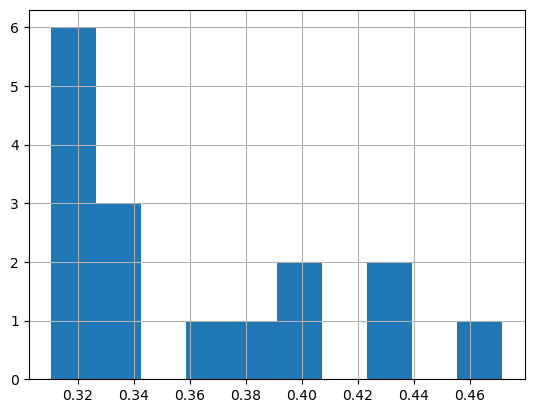
Comparisons#
You can pretty easily add new columns to a data frame (see below).
df["number 4"] = 4
df
subject_id | gaze | target | position | congruency | response | rt | correct_response | correct | slow | number 4 | |
---|---|---|---|---|---|---|---|---|---|---|---|
0 | example | left | H | left | congruent | m | 0.471526 | m | 1 | True | 4 |
1 | example | left | F | right | incongruent | y | 0.385041 | y | 1 | False | 4 |
2 | example | right | F | right | congruent | m | 0.315384 | y | 0 | False | 4 |
3 | example | right | H | left | incongruent | y | 0.327810 | m | 0 | False | 4 |
4 | example | right | H | right | congruent | m | 0.319960 | m | 1 | False | 4 |
5 | example | left | F | left | congruent | m | 0.359625 | y | 0 | False | 4 |
6 | example | left | F | left | congruent | y | 0.396527 | y | 1 | False | 4 |
7 | example | left | F | right | incongruent | m | 0.341541 | y | 0 | False | 4 |
8 | example | right | F | right | congruent | y | 0.423950 | y | 1 | True | 4 |
9 | example | left | H | left | congruent | m | 0.325600 | m | 1 | False | 4 |
10 | example | left | H | right | incongruent | y | 0.326414 | m | 0 | False | 4 |
11 | example | right | H | left | incongruent | m | 0.314234 | m | 1 | False | 4 |
12 | example | right | H | right | congruent | y | 0.430067 | m | 0 | True | 4 |
13 | example | right | F | left | incongruent | m | 0.310507 | y | 0 | False | 4 |
14 | example | left | H | right | incongruent | y | 0.394205 | m | 0 | False | 4 |
15 | example | right | F | left | incongruent | m | 0.335534 | y | 0 | False | 4 |
# Run this cell and try to understand what's going on here
df["slow"] = df["rt"] > 0.4
df
subject_id | gaze | target | position | congruency | response | rt | correct_response | correct | slow | |
---|---|---|---|---|---|---|---|---|---|---|
0 | example | left | H | left | congruent | m | 0.471526 | m | 1 | True |
1 | example | left | F | right | incongruent | y | 0.385041 | y | 1 | False |
2 | example | right | F | right | congruent | m | 0.315384 | y | 0 | False |
3 | example | right | H | left | incongruent | y | 0.327810 | m | 0 | False |
4 | example | right | H | right | congruent | m | 0.319960 | m | 1 | False |
5 | example | left | F | left | congruent | m | 0.359625 | y | 0 | False |
6 | example | left | F | left | congruent | y | 0.396527 | y | 1 | False |
7 | example | left | F | right | incongruent | m | 0.341541 | y | 0 | False |
8 | example | right | F | right | congruent | y | 0.423950 | y | 1 | True |
9 | example | left | H | left | congruent | m | 0.325600 | m | 1 | False |
10 | example | left | H | right | incongruent | y | 0.326414 | m | 0 | False |
11 | example | right | H | left | incongruent | m | 0.314234 | m | 1 | False |
12 | example | right | H | right | congruent | y | 0.430067 | m | 0 | True |
13 | example | right | F | left | incongruent | m | 0.310507 | y | 0 | False |
14 | example | left | H | right | incongruent | y | 0.394205 | m | 0 | False |
15 | example | right | F | left | incongruent | m | 0.335534 | y | 0 | False |
Group By#
If we have different groups within our data - in our case for example we have some congruent and some incongruent trials - we can use groupby
to get some grouped summary statistics for example, mean accuracy per group.
df.groupby("congruency")["correct"].mean()
congruency
congruent 0.625
incongruent 0.250
Name: correct, dtype: float64
df.groupby("congruency")["rt"].mean()
congruency
congruent 0.380330
incongruent 0.341911
Name: rt, dtype: float64
instead of computing the mean we can also use groupby to plot grouped histograms:
df.groupby("congruency")["rt"].hist()
congruency
congruent Axes(0.125,0.11;0.775x0.77)
incongruent Axes(0.125,0.11;0.775x0.77)
Name: rt, dtype: object
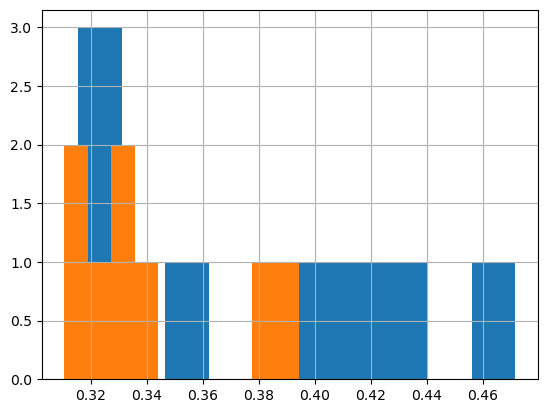
Visualisation with seaborn#
Let’s also go over some data visualisation using the widely used package seaborn
import seaborn as sns
Load an example dataset from seaborn
# Load an example dataset
tips = sns.load_dataset("tips")
tips
total_bill | tip | sex | smoker | day | time | size | |
---|---|---|---|---|---|---|---|
0 | 16.99 | 1.01 | Female | No | Sun | Dinner | 2 |
1 | 10.34 | 1.66 | Male | No | Sun | Dinner | 3 |
2 | 21.01 | 3.50 | Male | No | Sun | Dinner | 3 |
3 | 23.68 | 3.31 | Male | No | Sun | Dinner | 2 |
4 | 24.59 | 3.61 | Female | No | Sun | Dinner | 4 |
... | ... | ... | ... | ... | ... | ... | ... |
239 | 29.03 | 5.92 | Male | No | Sat | Dinner | 3 |
240 | 27.18 | 2.00 | Female | Yes | Sat | Dinner | 2 |
241 | 22.67 | 2.00 | Male | Yes | Sat | Dinner | 2 |
242 | 17.82 | 1.75 | Male | No | Sat | Dinner | 2 |
243 | 18.78 | 3.00 | Female | No | Thur | Dinner | 2 |
244 rows × 7 columns
# Create a visualization
sns.relplot(
data=tips,
x="total_bill", y="tip", col="time",
hue="smoker", style="smoker", size="size",
)
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
<seaborn.axisgrid.FacetGrid at 0x12aad4450>
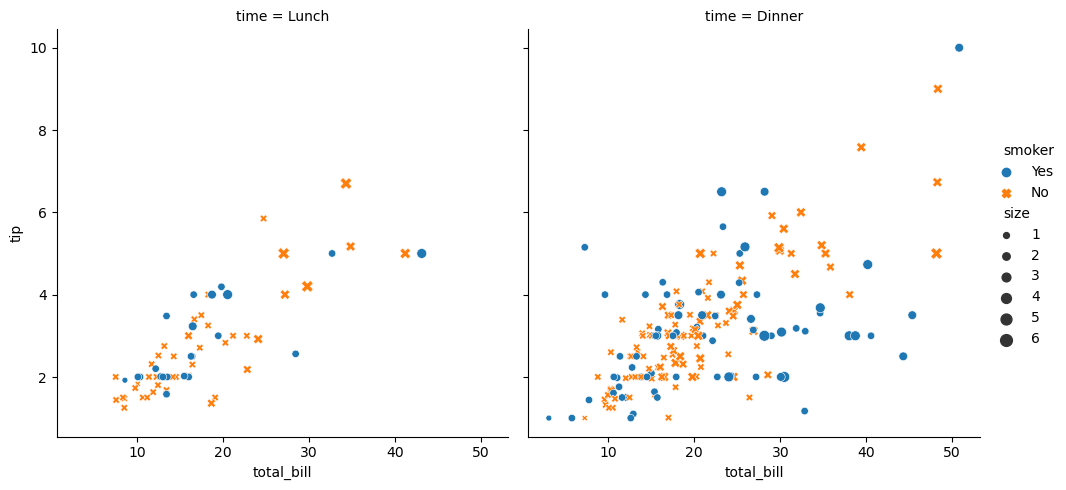
The default for relplot
are scatterplots as you can see. But you can easily change it to lineplots using the kind
argument. Lets try it on a new dataset that is better fitted for a lineplot: timeseries data
dots = sns.load_dataset("dots")
dots
align | choice | time | coherence | firing_rate | |
---|---|---|---|---|---|
0 | dots | T1 | -80 | 0.0 | 33.189967 |
1 | dots | T1 | -80 | 3.2 | 31.691726 |
2 | dots | T1 | -80 | 6.4 | 34.279840 |
3 | dots | T1 | -80 | 12.8 | 32.631874 |
4 | dots | T1 | -80 | 25.6 | 35.060487 |
... | ... | ... | ... | ... | ... |
843 | sacc | T2 | 300 | 3.2 | 33.281734 |
844 | sacc | T2 | 300 | 6.4 | 27.583979 |
845 | sacc | T2 | 300 | 12.8 | 28.511530 |
846 | sacc | T2 | 300 | 25.6 | 27.009804 |
847 | sacc | T2 | 300 | 51.2 | 30.959302 |
848 rows × 5 columns
sns.relplot(
data=dots, kind="line",
x="time", y="firing_rate", col="align",
hue="choice", size="coherence", style="choice",
facet_kws=dict(sharex=False),
)
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1119: FutureWarning: use_inf_as_na option is deprecated and will be removed in a future version. Convert inf values to NaN before operating instead.
with pd.option_context('mode.use_inf_as_na', True):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1119: FutureWarning: use_inf_as_na option is deprecated and will be removed in a future version. Convert inf values to NaN before operating instead.
with pd.option_context('mode.use_inf_as_na', True):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1119: FutureWarning: use_inf_as_na option is deprecated and will be removed in a future version. Convert inf values to NaN before operating instead.
with pd.option_context('mode.use_inf_as_na', True):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1119: FutureWarning: use_inf_as_na option is deprecated and will be removed in a future version. Convert inf values to NaN before operating instead.
with pd.option_context('mode.use_inf_as_na', True):
<seaborn.axisgrid.FacetGrid at 0x12b227b90>
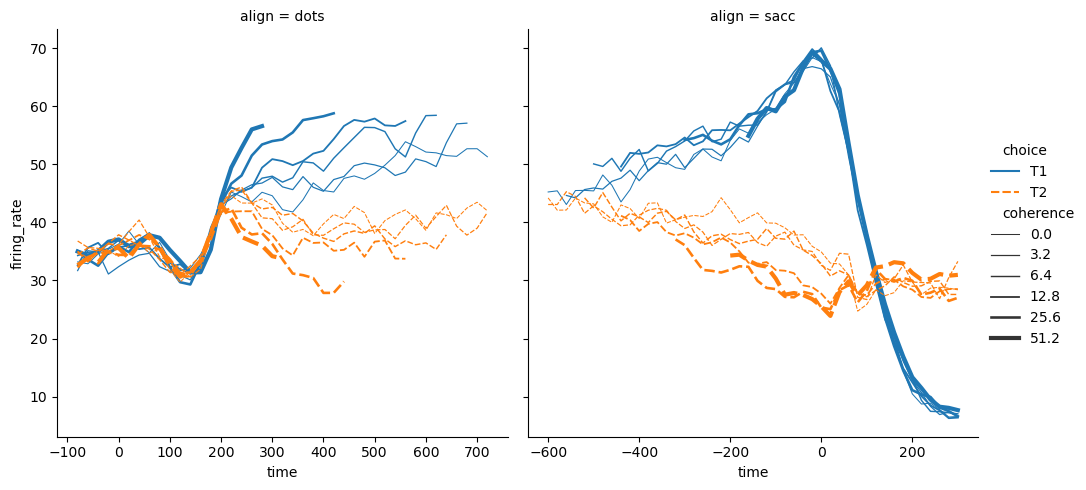
Including statistical estimates: means and confidence intervals
fmri = sns.load_dataset("fmri")
sns.relplot(
data=fmri, kind="line",
x="timepoint", y="signal", col="region",
hue="event", style="event",
)
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1119: FutureWarning: use_inf_as_na option is deprecated and will be removed in a future version. Convert inf values to NaN before operating instead.
with pd.option_context('mode.use_inf_as_na', True):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1119: FutureWarning: use_inf_as_na option is deprecated and will be removed in a future version. Convert inf values to NaN before operating instead.
with pd.option_context('mode.use_inf_as_na', True):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1119: FutureWarning: use_inf_as_na option is deprecated and will be removed in a future version. Convert inf values to NaN before operating instead.
with pd.option_context('mode.use_inf_as_na', True):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1119: FutureWarning: use_inf_as_na option is deprecated and will be removed in a future version. Convert inf values to NaN before operating instead.
with pd.option_context('mode.use_inf_as_na', True):
<seaborn.axisgrid.FacetGrid at 0x12b285790>
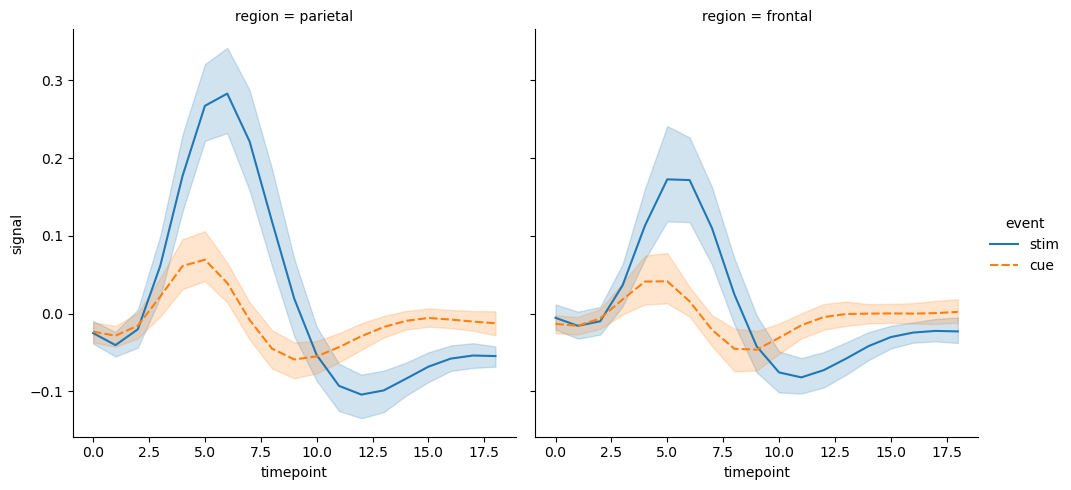
You can also easily include a linear regression model and its uncertainty using lmplot
:
sns.lmplot(data=tips, x="total_bill", y="tip", col="time", hue="smoker")
<seaborn.axisgrid.FacetGrid at 0x12b494110>
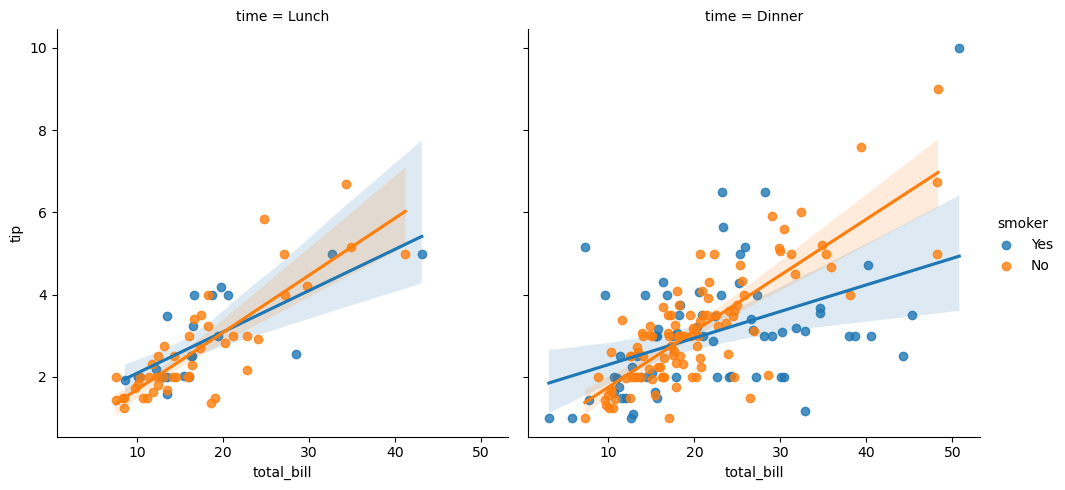
Exercises
1.1 create a boxplot with seaborn for our gaze cue data where you plot reaction time by congruency with the colors white and blue
sns.boxplot(x="congruency", y="rt",
palette=["w", "b"],
data=df)
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
<Axes: xlabel='congruency', ylabel='rt'>
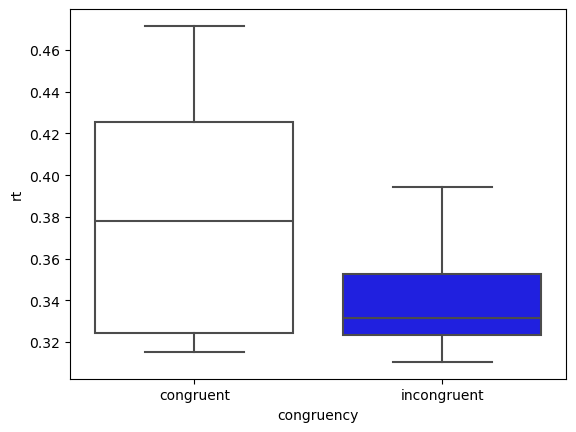
1.2 Do the same with a barplot, this time using colors of your choice
sns.set_theme(style="ticks", palette="pastel")
sns.barplot(data = df, x="congruency", y="rt",
palette=["y", "b"], errorbar = None)
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
/Users/aylinkallmayer/anaconda3/envs/pfp_2023-24/lib/python3.11/site-packages/seaborn/_oldcore.py:1498: FutureWarning: is_categorical_dtype is deprecated and will be removed in a future version. Use isinstance(dtype, CategoricalDtype) instead
if pd.api.types.is_categorical_dtype(vector):
<Axes: xlabel='congruency', ylabel='rt'>
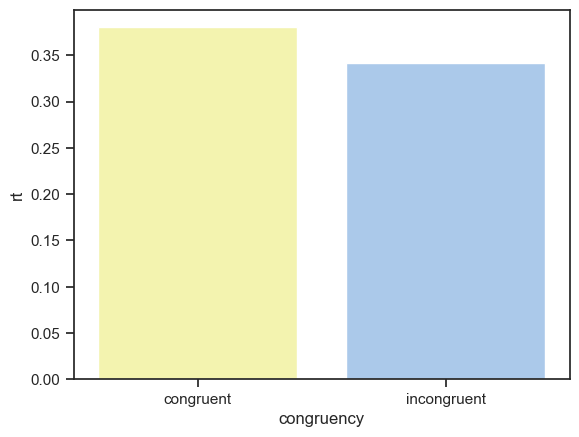