New Year recap#
In today’s session, we will do a recap of the concepts that most of you indicated needed some more practicing. This includes looking back at for and while loops as well as functions. Even if you feel pretty confident already, continued practice on new problems is always a good way to solidify your knowledge. If you feel like you’re lagging behind and you don’t understand these concepts at all, please go back to the previous Python III notebook and keep revising these topics. Today, we will do some live problem solving and work on more example exercises.
For Loops#
First, let’s look at for loops again. A for loop always requires some iterable - a collection of objects. What are some iterables you have learned about already?
it_string = "this is an iterable with many things inside"
it_string_short = "string"
it_list = [1,2,3]
it_range = range(5)
it_dictionary = {
"first": 0,
"second": 1,
"third": 2
}
it_tuple = (4,5,6)
it_list_of_iterables = [it_string, it_string_short, it_list, it_dictionary, it_tuple]
We can loop through elements of an iterable with for loops
for s in it_string_short: # defining var (s) and iteraterable (it_string)
print(s) # body of the loop: what should happen with each iteration?
s
t
r
i
n
g
for l in it_list:
print(l)
1
2
3
for r in it_range:
print(r+1)
1
2
3
4
5
for item in it_dictionary.values():
print(item)
0
1
2
for it in it_list_of_iterables:
print(type(it), it)
<class 'str'> this is an iterable with many things inside
<class 'str'> string
<class 'list'> [1, 2, 3]
<class 'dict'> {'first': 0, 'second': 1, 'third': 2}
<class 'tuple'> (4, 5, 6)
List comprehensions#
Imagine you have some iterable (e.g., list) and you want to loop through each item of this iterable with the goal to at the end have a list, that stores the output of the loop body from each iteration. you could do it like this:
it_list = [1,2,3,4,5,6,7,8,9]
outcome_list = []
for i in it_list:
new_i = i/100
outcome_list.append(new_i)
print(outcome_list)
[0.01, 0.02, 0.03, 0.04, 0.05, 0.06, 0.07, 0.08, 0.09]
The purpose of list comprehensions is to reduce the number of individual steps required to produce this specific goal, by making it a “one liner”
it_list = [1,2,3,4,5,6,7,8,9]
outcome_list = [i/100 for i in it_list]
print(outcome_list)
[0.01, 0.02, 0.03, 0.04, 0.05, 0.06, 0.07, 0.08, 0.09]
What if we wanted to include a conditional statement in our list comprehension? This is possible for simple if-else statements:
outcome_list = [i/100 for i in it_list if i<5]
print(outcome_list)
[0.01, 0.02, 0.03, 0.04]
If we want to include what should happen if the statement evaluates to false (basically in the else
case), we need to move it to the front of the list comprehension, before the for x in y
outcome_list = [i/100 if i < 5 else i for i in it_list]
print(outcome_list)
[0.01, 0.02, 0.03, 0.04, 5, 6, 7, 8, 9]
Exercise
The secret number game:
Use the random.randint(lower_limit, upper_limit)
function to generate a secret number between the specified range and store it in a variable called secret_number
.
Allow the player to make multiple guesses by setting max_attempts
to the number of guesses you want to allow.
Loop through the number of guesses you allow and provide feedback for whether the guessed number was correct, too high or too low.
End the game when the player correctly guesses the secret number or after a certain number of attempts.
In order to submit a guess for each attempt you can use int(input(f"\nAttempt {attempt}/{max_attempts}: Enter your guess: "))
and assign it to a variable called guess
.
Hints:
Use if, elif, and else statements to provide feedback on the player’s guess.
Include a break statement to exit the loop when the player guesses correctly.
Feel free to customize the range, the number of attempts, and any other aspects of the game to make it more engaging!
import random
# Set the range for the secret number
lower_limit = 1
upper_limit = 20
# Generate a random secret number
secret_number = random.randint(lower_limit, upper_limit)
# Set the maximum number of attempts
max_attempts = 3
# print some welcome statement that tells the player the lower and upper limits of their guesses as well as the number of guesses available
print("the lower limit is {} & the upper limit is {}. You have a maximum of {} attempts".format(lower_limit,upper_limit,max_attempts))
# create a for loop that asks for a guess, provides feedback on the guess and exits when the game ends (either correct guess or running out of nr of attempts)
for attempt in range(max_attempts):
guess = int(input(f"\nAttempt {attempt}/{max_attempts}: Enter your guess: "))
if guess == secret_number:
print("Congratulations! You won the game by guessing the correct number")
break
elif guess < secret_number:
print("Too low! Guess again")
else:
print("Too high! Guess again")
else:
print(f"You ran out of attempts, better luck next time. The secret number was {secret_number}")
the lower limit is 1 & the upper limit is 20. You have a maximum of 3 attempts
Attempt 0/3: Enter your guess: 10
Too high! Guess again
Attempt 1/3: Enter your guess: 5
Too high! Guess again
Attempt 2/3: Enter your guess: 2
Too high! Guess again
You ran out of attempts, better luck next time. The secret number was 1
Exercise
Grade calculator:
Create a variable called num_students
and assign it 20.
Create a variable called grades
and fill it with random numbers between 1 and 6 for each student via a list comprehension.
Calculate and print the average score across students.
import numpy as np
# number of students
num_students = 20
# generate grades for each student
grades = [random.randint(1, 6) for i in range(num_students)]
print(grades)
# print average grade
print(np.mean(grades))
[2, 2, 2, 2, 5, 1, 2, 3, 4, 6, 4, 6, 4, 5, 5, 6, 6, 3, 4, 1]
3.65
Now use another list comprehension to create a new list called passed
that only includes grades better or equal to 4 and calculate the mean over the new list.
# your solution here
passed = [i for i in grades if i<=4]
print(passed)
print(np.mean(passed))
[2, 2, 2, 2, 1, 2, 3, 4, 4, 4, 3, 4, 1]
2.6153846153846154
Functions#
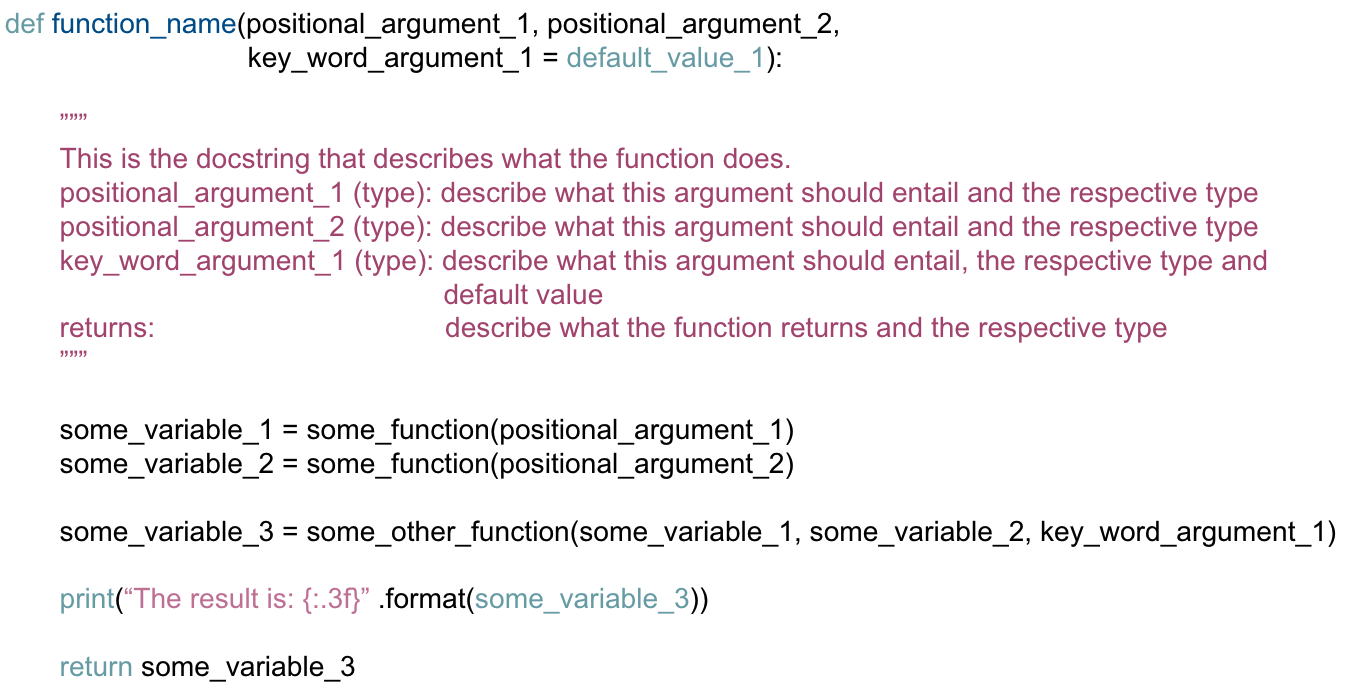
Exercise
you will create a simple text-based adventure game called “Escape the Dungeon.” The goal is to guide the player through a series of rooms, each presenting a unique challenge or obstacle. The player must make choices to overcome these challenges and successfully escape the dungeon.
You need to create two functions: room_challenge
and escape_dungeon
.
The room_challenge
function should take in one positional argument room_number
. First, it should print the room number and a prompt that says “there is a challenge you have to complete”. Then, you should create a variable success
that randomly evaluates to True
or False
. If the challenge went successful, print a congratulations statement and return True
. If not, print a statement that says the challenge failed and return False
.
The escape_dungeon
function should take in one positional argument num_rooms
. First, it should print a prompt that welcomes the player and tells them how many rooms awaits them. Then, for each room, call the room_challenge
function. If the challenge was successful, let the player know they successfully cleared the room. If not, exit the game and print a statement letting the play know they lost the game. If all rooms were cleared successfully, print a statement letting the play know they escaped the dungeon.
import random
# define the first function
def room_challenge(room_number):
print(f"This is room number {room_number+1}! There is a challenge to complete!")
success = random.choice([True, False])
if success:
print("Congratulations! \nyou completed this challenge")
return True
else:
print("Unfortunately, you failed this challenge")
return False
# define the second function
def escape_dungeon(num_rooms):
print("Welcome Player! {} rooms await you \n ".format(num_rooms))
for room_nr in range(num_rooms):
successful = room_challenge(room_nr)
if successful:
print("you successfully cleared this room! Onto the next one. \n ")
else:
print("Sorry, better luck next time, you failed the game!")
break
else:
print("Congratulations! You won the game")
# call the function to mock "play" the game
escape_dungeon(3)
Welcome Player! 3 rooms await you
This is room number 1! There is a challenge to complete!
Congratulations!
you completed this challenge
you successfully cleared this room! Onto the next one.
This is room number 2! There is a challenge to complete!
Congratulations!
you completed this challenge
you successfully cleared this room! Onto the next one.
This is room number 3! There is a challenge to complete!
Congratulations!
you completed this challenge
you successfully cleared this room! Onto the next one.
Congratulations! You won the game